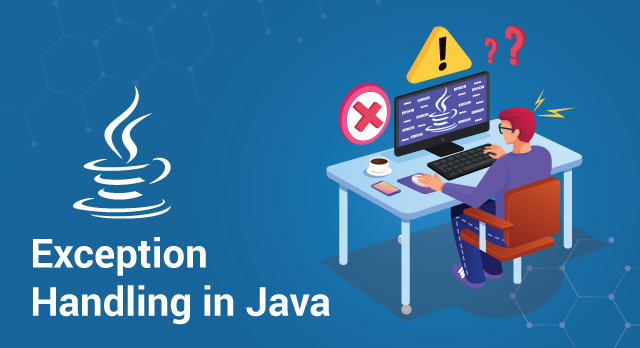
In the unpredictable world of Java programming, exceptions are like unexpected storms that can abruptly disrupt the flow of your code. While we can't control when these events occur, we can equip ourselves with exception handling techniques to navigate them gracefully, ensuring our applications remain robust and user-friendly.
Here's a comprehensive guide to mastering exception handling in Java:
1. Understanding Exceptions:
- Exceptions signal abnormal events or errors that interrupt normal execution.
- They can arise from various sources, including:
- Attempting to divide by zero
- Trying to access a file that doesn't exist
- Converting invalid data types
2. The try-catch
Block: Your Lifejacket
- The core mechanism for handling exceptions is the
try-catch
block:
try { // Code that might throw exceptions} catch (ExceptionType exceptionObject) { // Code to handle the exception}
- Enclose potentially risky code within the
try
block. - Catch specific exception types using
catch
blocks.
3. Essential Keywords:
throw
: Used to manually throw an exception.throws
: Declares that a method might throw an exception, deferring handling to the caller.
4. Common Exception Types:
IOException
: For file-related issuesNumberFormatException
: For invalid number conversionsArithmeticException
: For arithmetic errors like division by zeroNullPointerException
: For attempting to use a null referenceIllegalArgumentException
: For invalid argument values
5. Advanced Techniques:
finally
Block: Code within afinally
block always executes, even if an exception occurs or areturn
statement is encountered, ensuring critical actions like closing resources.try-with-resources
Statement: Automatic resource management for cleaner code.- Custom Exceptions: Define your own exception types for specific error scenarios.
Best Practices for Exception Handling:
- Use specific exception types: Catch more specific exceptions for targeted handling.
- Log exceptions for debugging: Record details for troubleshooting.
- Provide meaningful error messages: Inform users about issues clearly.
- Handle exceptions gracefully: Avoid program crashes and guide users through recovery.
By effectively implementing exception handling, you'll create more robust, resilient, and user-friendly Java applications that can anticipate and navigate unexpected challenges with grace. Embrace this vital tool to enhance the stability and dependability of your code!